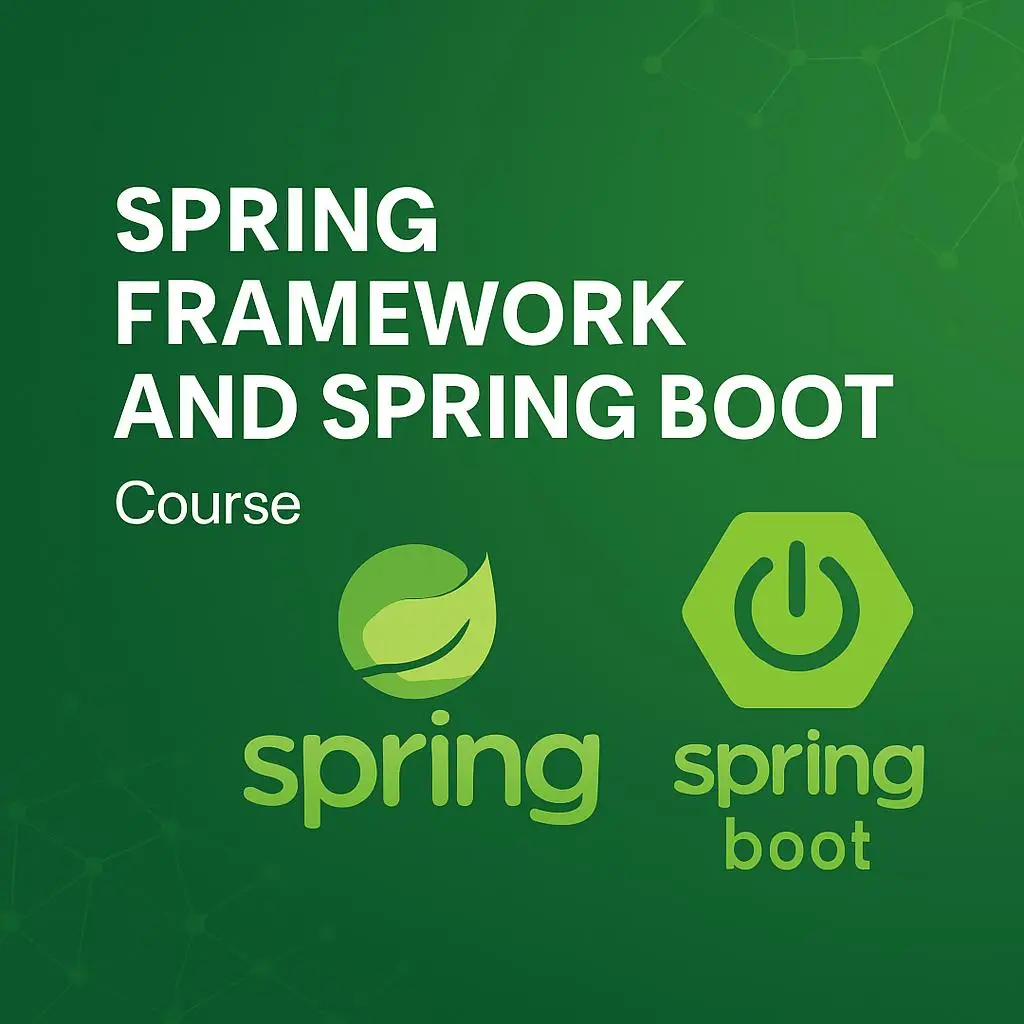
Index
Master Spring Boot
Master Spring Boot with our comprehensive course designed specifically for developers looking to enhance their Java skills. Gain hands-on experience building scalable web applications from scratch.
Our practical course guides you through key topics such as creating CRUD applications, executing unit tests, documenting RESTful APIs with Swagger, and applying Behavior-Driven Development (BDD) with Cucumber.
What You’ll Learn in Our Master Spring Boot Course
By the end of this course, you’ll be able to Master Spring Boot and confidently apply your skills in real-world projects.
- Develop full-featured web applications using Spring Boot.
- Implement effective CRUD operations.
- Perform automated unit tests seamlessly.
- Create clear API documentation with Swagger integration.
- Improve your workflow using Cucumber for efficient BDD.
Who is This Spring Boot Course For?
Whether you’re an aspiring Java developer, a student seeking to gain competitive skills, or a professional aiming to upgrade your current Java expertise, mastering Spring Boot is essential for your career growth. This course will provide you with the knowledge and confidence needed to develop high-quality Java applications efficiently.
By the end of this course, you’ll possess the practical knowledge required to build scalable and maintainable applications using industry-standard methodologies.
Why Developers Opt to Master Spring Boot for Modern Java Development?
Spring Boot is the most widely used Java framework globally, praised for its simplicity, efficiency, and productivity. Becoming proficient in Spring Boot significantly increases your professional value, making you competitive in the rapidly evolving software development market.
Mastering Spring Boot offers several compelling advantages for Java developers:
- Enhanced Productivity: Spring Boot’s opinionated defaults and embedded servers allow developers to set up and run applications quickly, reducing the need for extensive configuration.
- Microservices Architecture: Spring Boot facilitates the development of microservices, enabling the creation of modular and scalable applications that are easier to manage and deploy.
- Comprehensive Ecosystem: With a vast array of extensions and third-party libraries, Spring Boot provides tools to build almost any application imaginable, from web apps to complex data processing systems.
- Industry Demand: Given its widespread adoption, proficiency in Spring Boot is a valuable skill in the job market, opening opportunities in various sectors that rely on Java technologies.
Course Benefits
Companies worldwide are looking for developers who can Master Spring Boot to build modern, efficient Java applications.
- Practical instruction tailored for real-world scenarios.
- Immediate applicability to industry-standard Java projects.
- Increased marketability as a skilled Java developer.
Don’t miss this opportunity to Master Spring Boot and boost your career as a Java developer.
Summary
If you would like more information, you can visit the official Spring Boot documentation.
Module 1 – Requirements, project and MongoDB setup
Unit 1 – Requirements
All the requirements we need:
- Java Developer Kit
- Git
- IntelliJ
- MongoDB Compass
Unit 2 – Project & MongoDB collection creation
- Spring project creation
- MongoDB collection
Module 2 – Developing our first CRUD of products
Unit 3 – CRUD
- Data Transfer Objects definition:
- We will create different Java classes to define the objects that will be received and returned from the service.
- Entity definitions:
- Those Java classes are going to be used just to access the database.
- CRUD endpoints:
- Get endpoint: This endpoint will be used to retrieve the data using different filters. We are going to be able to:
- Retrieve all data.
- Retrieve data filtered by different fields, such as code, name, and description.
- Put endpoint: It will be used to add new collections to MongoDB.
- Post endpoint: This is for updating collections that already exist on DB.
- Delete: Used for removing collections on DB.
- Get endpoint: This endpoint will be used to retrieve the data using different filters. We are going to be able to:
- Create a MongoDB and our first collection.
- Create a basic README.md file.
- Exception handling: We are going to see how to control different exceptions.
- Mapping DTOs and Entities.
- PostMan collection: We are going to add a Postman collection to make it easier for you to try different endpoints locally.
Module 3 – Unit Testing with JUnit and Mockito
Unit 4 – Unit Testing
- Unit testing
- JUnit and Mockito
- Arrange, Act and Assert
- Total coverage of code
- doThrow vs thenThrow
- Improvements
Unit 5 – Improvements & Transactions
- Code improvements
- Transactional annotation
Unit 6 – Swagger
- Documentation
- Interactive API Testing
- Contracts
Unit 7 – Actuator
- Exposing built-in endpoints
- Health
- Info
Unit 8 – Karate
- Integration & Automated Testing
Unit 9 – Cucumber
- BDD (Behavior-Driven Development)